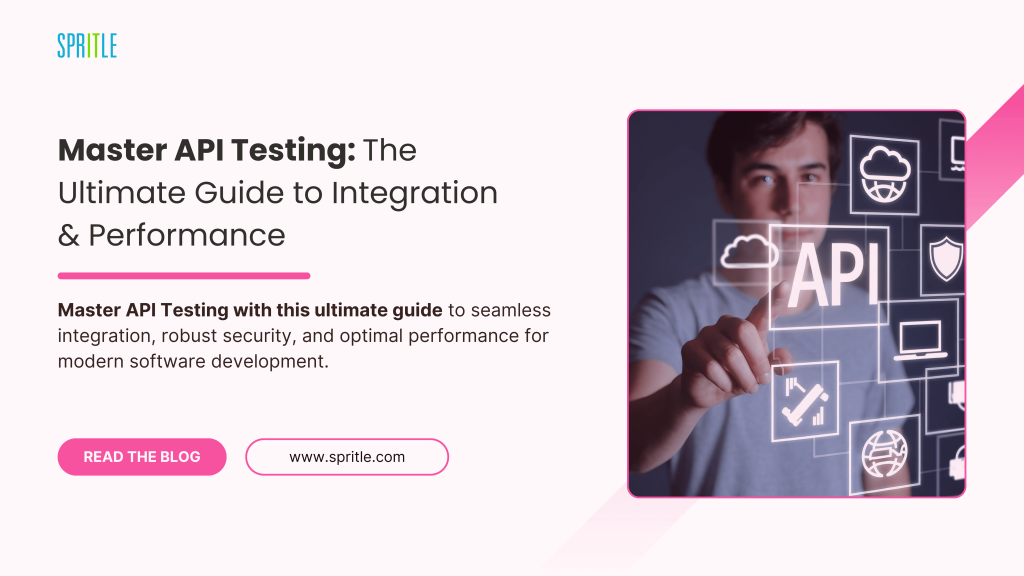
In at the moment’s digital panorama, APIs (Software Programming Interfaces) play a vital position in connecting techniques, purposes, and providers. With the rising reliance on APIs, making certain their reliability, safety, and efficiency via rigorous testing is crucial. This information explores the core parts of an efficient API testing methodology, masking completely different testing sorts, greatest practices, and ideas for implementation.
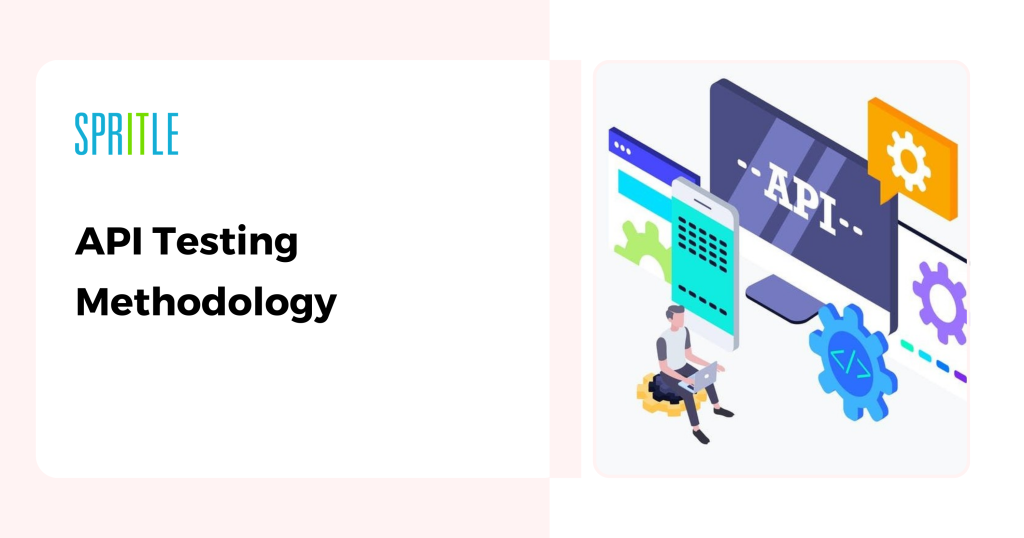
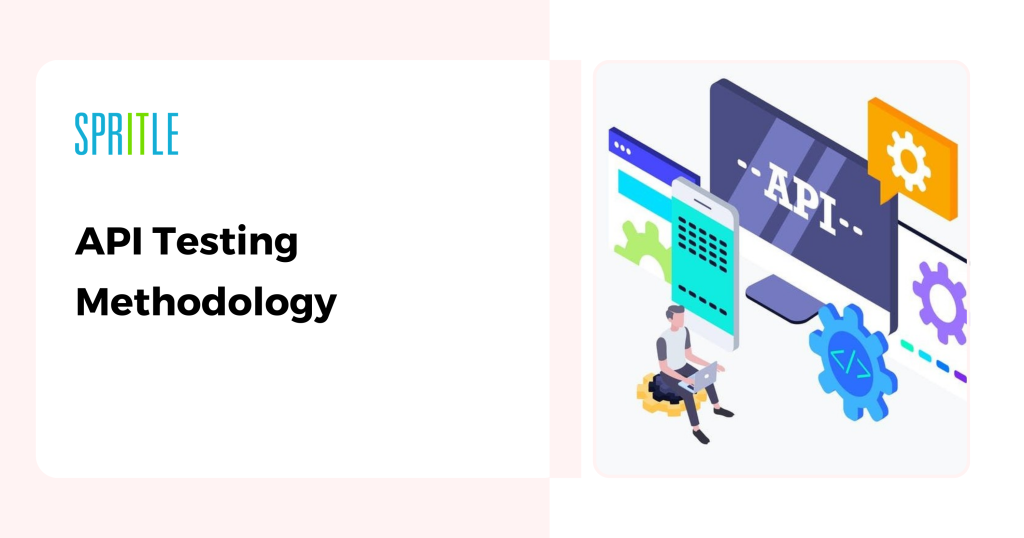
1. Introduction to API Testing
API testing includes testing APIs straight to make sure they fulfill anticipated performance, reliability, efficiency, and safety. In contrast to UI testing, API testing bypasses the person interface and straight validates the appliance logic on the API layer. API testing instrument that helps builders and testers create, check, and doc APIs. It has a user-friendly interface that helps a wide range of HTTP strategies, authentication sorts, and information codecs.
Why API Testing Issues
APIs act as intermediaries for purposes to speak with each other. Correct API testing ensures seamless integration, environment friendly information exchanges, and safe data flows throughout techniques.Testing your API is crucial to the correct integration and supply of high quality software program and product. In contrast to UI testing, API automation testing is designed to resist the brief launch cycles and frequent adjustments that happen whereas utilizing greatest practices for software program improvement and IT operations.
2. Sorts of API Testing:
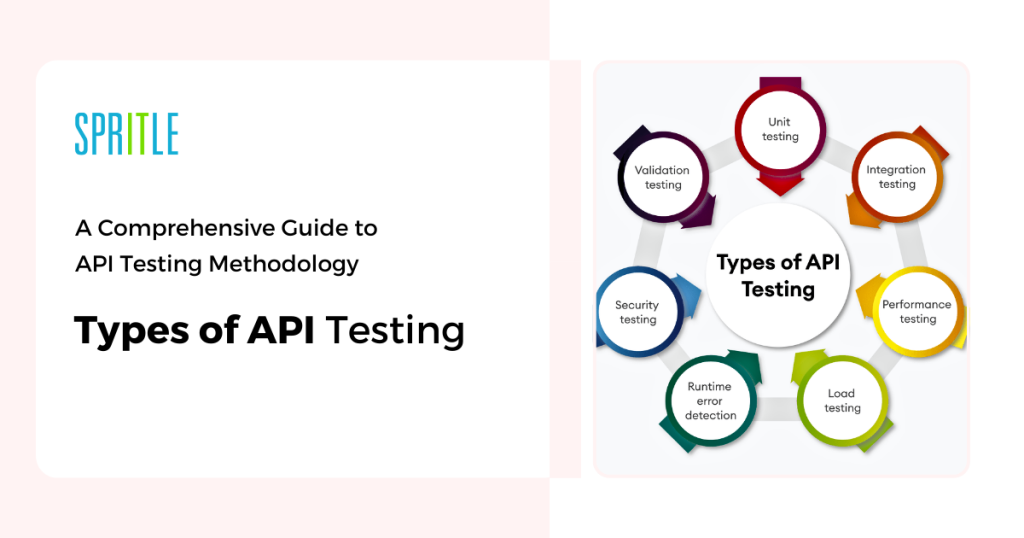
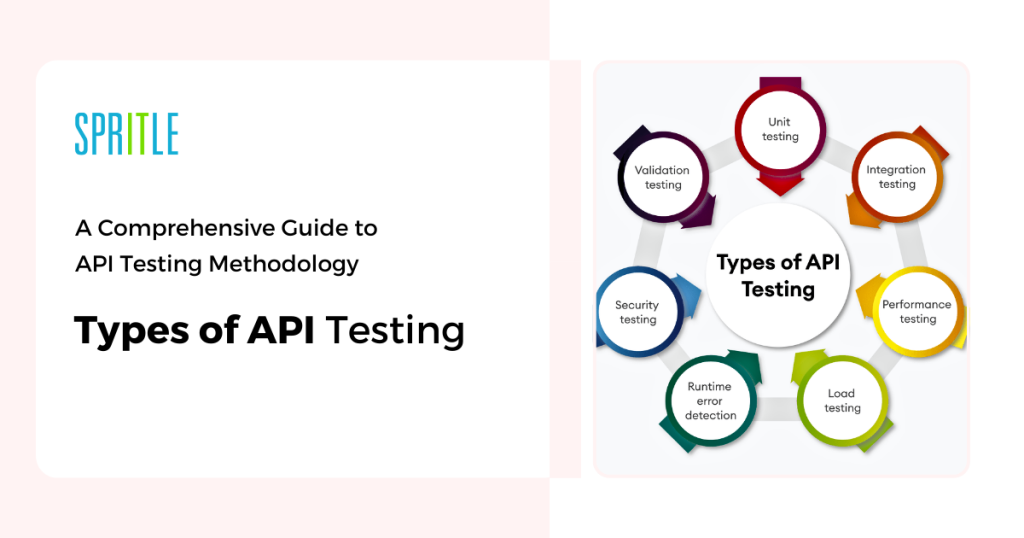
Understanding the several types of API testing helps testers deal with particular points inside an API’s performance:
Purposeful Testing: Validates that the API capabilities in accordance with its specification, specializing in response information accuracy and correctness.Integration Testing: Ensures the API accurately interacts with different APIs, parts, and providers.Efficiency Testing: Efficiency testing is a testing measure that evaluates the pace, responsiveness and stability of a pc, community, software program program or gadget below a workload.Load Testing: Checks the API below excessive volumes of requests to evaluate its stability, efficiency, and response instances.Runtime Error Detection: Runtime error detection is a software program verification methodology that analyzes a software program software because it executes and reviews defects which are detected throughout that execution.Safety Testing: Identifies potential vulnerabilities, making certain the API is protected towards unauthorized entry and information breaches.Validation Testing: Validation testing is the method of assessing a brand new software program product to make sure that its efficiency matches shopper wants.Reliability Testing: Verifies the API’s means to perform reliably over time, notably below fluctuating hundreds.Finish-to-Finish Testing: Checks the total performance of the API throughout the context of your complete software workflow.
3. API Testing Course of:
A structured method to API testing helps streamline efforts and maximize check protection. Right here’s a breakdown of the standard course of:
Step 1: Outline the Scope and Necessities
Perceive Necessities: Collect detailed API specs, reminiscent of request parameters, response codecs, and anticipated behaviour.Set Check Goals: Outline what you wish to obtain with testing (e.g., validating performance, making certain safety, checking load dealing with).
Step 2: Set up Check Instances
Create particular check instances based mostly on the necessities, together with optimistic and damaging check situations. Check instances ought to cowl:
Legitimate and invalid requestsBoundary conditionsDifferent information typesError dealing with situations
Step 3: Select Testing Instruments
Choose instruments that fit your testing wants and surroundings. Some in style API testing instruments embody:
Postman: Nice for handbook and automatic API testing.JMeter: For load and efficiency testing.SoapUI: For complete purposeful and safety testing.Relaxation-Assured: A Java-based library for automated testing of REST APIs.Newman: A command-line instrument that works effectively with Postman for CI/CD.
Step 4: Check Execution
Run Checks: Execute the checks in a managed surroundings, simulating real-life API interactions.Log Outcomes: Accumulate responses, doc errors, and measure response instances to make sure the API meets outlined expectations.
Step 5: Validation and Verification
Use assertions to validate:
Response Standing Codes: Guarantee appropriate standing codes are returned (e.g., 200 for achievement, 404 for not discovered, 500 for server errors).
Response Physique: Validate the construction and content material of the response physique, checking that fields, information sorts, and values match expectations.Headers and Authentication: Test required headers and validate authentication mechanisms.
4. Introduction to the Layered Structure:
Trendy purposes are sometimes designed with a three-layer structure, usually represented as follows:
Presentation Layer: The user-facing interface, which may very well be an online or cell software.Enterprise Layer: The core logic that processes requests and manages the appliance’s capabilities.Database Layer: The storage layer, which handles the appliance’s information administration.
This layered construction permits for scalability, modularity, and simpler upkeep. Let’s dive into how every layer capabilities and use Postman to check APIs that work together with every layer.
Diagram of Layered API Testing
Right here’s a pattern visible diagram for example the movement and testing at every layer:
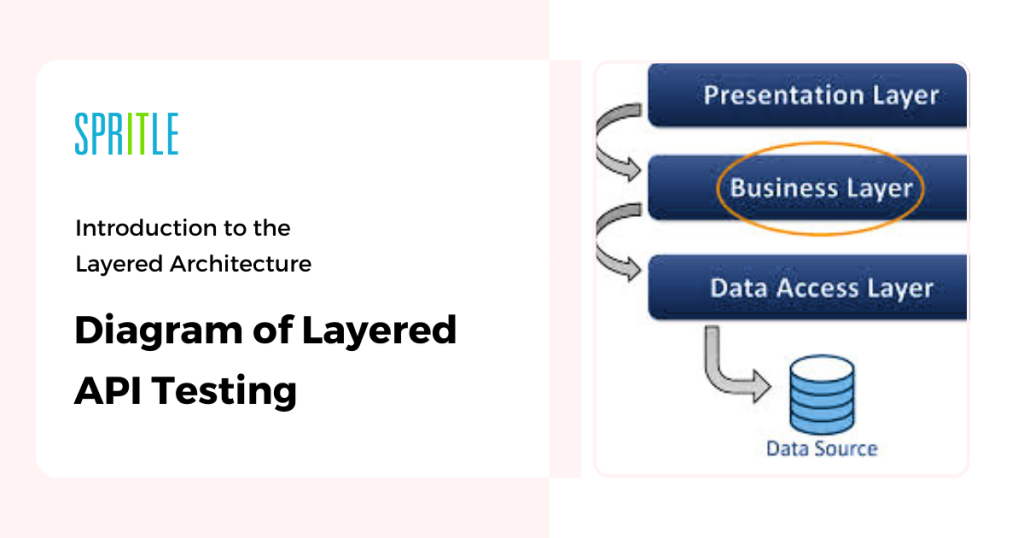
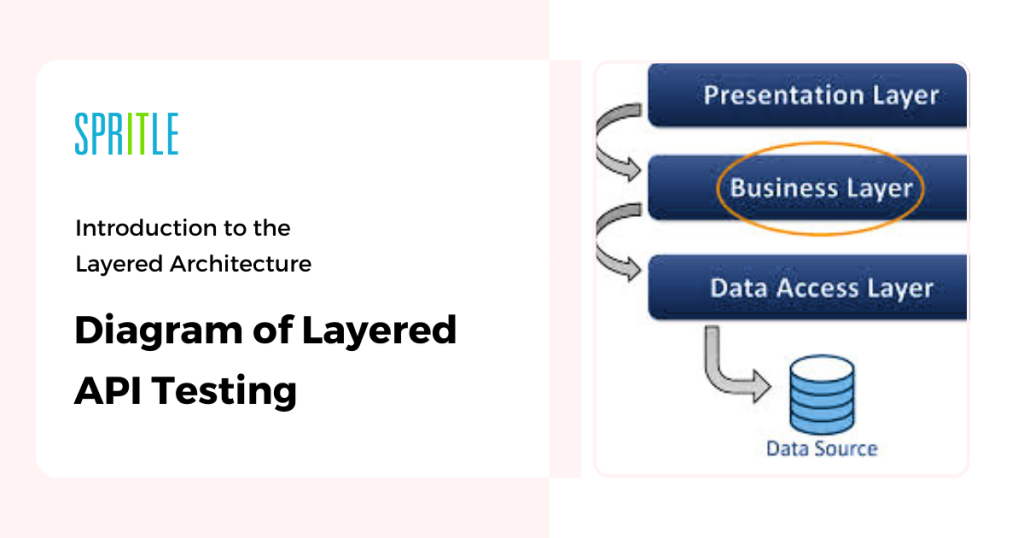
How the Layers Work together in API Testing?
Presentation Layer: Receives inputs (e.g., login, submit kind), sends them to the Enterprise Layer.Enterprise Layer: Processes the inputs, applies enterprise guidelines, interacts with the Database Layer as wanted.Database Layer: Shops or retrieves information based mostly on the Enterprise Layer’s requests.
i) Presentation Layer
What’s the Presentation Layer?
The Presentation Layer is the place customers work together with the appliance. It often consists of a graphical person interface (GUI), reminiscent of an online or cell app. This layer communicates with the Enterprise Layer via API requests.
Testing the Presentation Layer with Postman
Though Postman is primarily used for backend API testing, you’ll be able to nonetheless simulate how the Presentation Layer interacts with the Enterprise Layer. For instance:
Consumer Eventualities: Simulate person actions like logging in, viewing information, or submitting varieties by sending requests to the API endpoints that the UI would name.Endpoint Verification: Test that the endpoints utilized by the UI return the right information in response to completely different requests.
Instance
In Postman:
Create a request that mimics a person login (POST /login).Embrace parameters like username and password within the request physique.Test the response standing and response physique to make sure it matches what the UI would show (e.g., success message or person information).
ii)Enterprise Layer
What’s the Enterprise Layer?
The Enterprise Layer comprises the core logic that processes person inputs, manages interactions, and enforces enterprise guidelines. When an API request is acquired, it’s handed to the Enterprise Layer, which processes the logic and returns a response.
Testing the Enterprise Layer with Postman
Within the Enterprise Layer, you’ll be able to check completely different situations to make sure the logic behaves as anticipated. This usually contains:
Purposeful Testing: Guaranteeing the logic within the Enterprise Layer capabilities as anticipated. As an example, a “calculate low cost” API ought to accurately apply reductions based mostly on guidelines within the Enterprise Layer.Validation Testing: Test enter validations by sending numerous information sorts and edge instances.Error Dealing with: Ship invalid information to confirm that the API gracefully handles errors and returns the anticipated error codes and messages.
Instance
In Postman:
Ship a POST request to /applyDiscount, together with a payload with product and low cost particulars.Test if the response precisely displays the anticipated low cost or returns an applicable error if the enter is invalid.
iii)Database Layer
What’s the Database Layer?
The Database Layer is chargeable for information storage, retrieval, and administration. It ensures that the appliance’s information is saved and might be accessed by the Enterprise Layer when wanted.
Testing the Database Layer with Postman
Knowledge Integrity Testing: Carry out create, learn, replace, and delete (CRUD) operations by way of API requests and ensure the info displays these adjustments precisely.Question Validation: Be certain that API responses embody the right information based mostly on request parameters.Publish-Request Validation: After sending a request (e.g., making a person), confirm that the database has been up to date by sending a GET request to retrieve the info.
Instance
In Postman:
Ship a POST request to /createUser, together with person information within the request physique.After sending the request, ship a GET request to /getUser with the person ID.Confirm that the response matches the info you initially despatched.
5. API Testing Overview:
API (Software Programming Interface) testing focuses on verifying that completely different software program parts can talk successfully. Within the context of client-server structure, an API permits the consumer (reminiscent of a frontend software or cell app) to ship requests to a server, which then processes these requests and sends again responses.
1. Consumer-Server Structure:
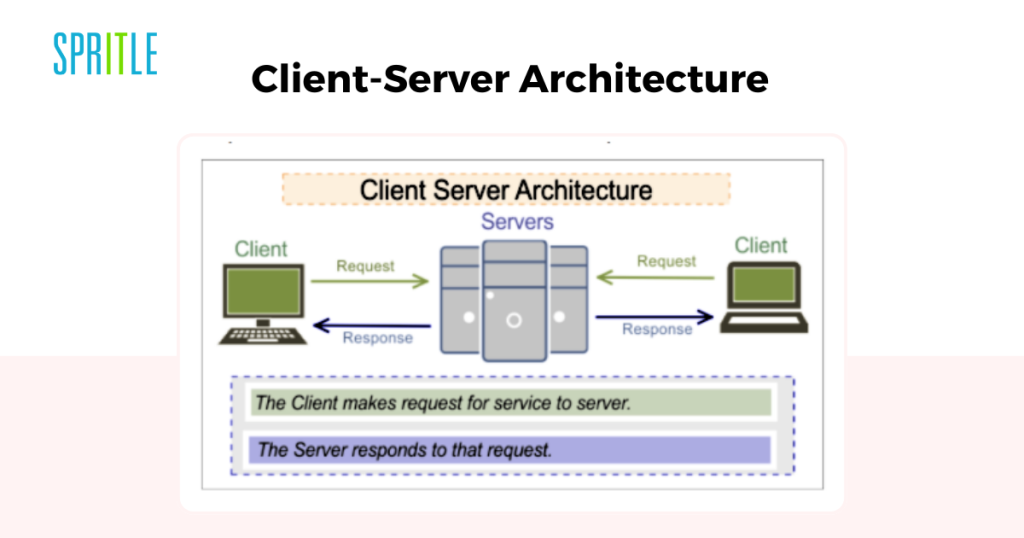
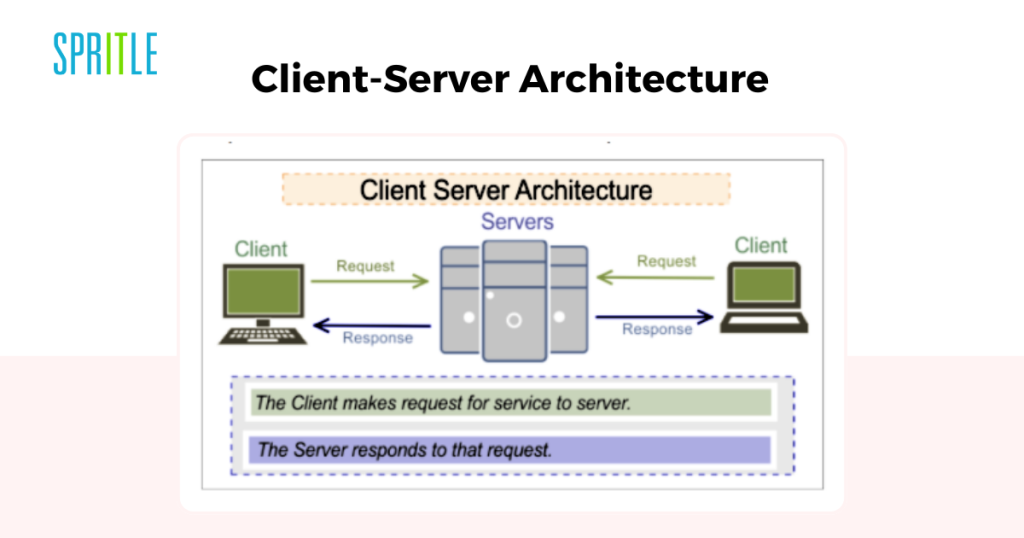
In client-server structure:
The Consumer (e.g., an online browser or cell app) initiates requests, often via an API.The Server (backend server or database server) processes these requests, executes any vital enterprise logic, and returns a response.
In API testing, you act because the consumer utilizing a instrument like Postman to ship requests to the API, simulating how an actual software would work together with the backend server.
2. Sorts of API Requests and Clarification:
APIs generally use HTTP requests, that are grouped into 4 main sorts:
GET : Retrieves information from the server.
Instance: A GET request to /customers would retrieve a listing of all customers.
POST : Sends information to the server to create a brand new useful resource.
Instance: A POST request to /customers with person particulars within the request physique creates a brand new person within the database.
PUT : Updates an current useful resource on the server.
Instance: A PUT request to /customers/1 with up to date information adjustments particulars of the person with ID 1.
DELETE : Removes a useful resource from the server.Instance: A DELETE request to /customers/1 would delete the person with ID 1.
3. Instance of API Request and Response
Let’s stroll via a primary API request and response movement:
To combine with the ReqRes API ( you need to use Postman to ship requests, work together with its endpoints and get the response. I’ll information you thru establishing a number of instance requests, reminiscent of GET, POST, PUT, and DELETE strategies.
Step 1: Open Postman
Open Postman in your laptop.
Step 2: Select an Endpoint
ReqRes presents a number of endpoints for testing, together with:
Step 3: Set Up and Check Requests in Postman
1. GET Request to Checklist Customers
In Postman:
Set the strategy to GET.Enter the URL: Ship.View the response, which ought to comprise a JSON array of customers.
2. POST Request to Create a Consumer
Physique: json
In Postman:
Set the strategy to POST.Enter the URL: to the Physique tab, choose uncooked, and set the sort to JSON.Enter the JSON physique above.Click on Ship.The response ought to embody the main points of the newly created person together with an ID and timestamp.
3. PUT Request to Replace a Consumer
In Postman:
Set the strategy to PUT.Enter the URL: to the Physique tab, choose uncooked, and set the sort to JSON.Enter the JSON physique above.Click on Ship.The response ought to present the up to date person data with the present timestamp.
4. DELETE Request to Take away a Consumer
In Postman:
Set the strategy to DELETE.Enter the URL: Ship.It is best to obtain a 204 No Content material response, indicating the person was deleted.
Conclusion:
API testing is a foundational side of recent software program improvement, making certain that APIs are purposeful, safe, and performant. By following a structured methodology, leveraging the correct instruments, and implementing greatest practices, you’ll be able to optimize your API testing course of. Because the demand for sturdy APIs continues to develop, investing time and sources in API testing will enhance your product’s reliability, person expertise, and long-term success.